Frontend Applications
You can build front-end applications by using our React and TypeScript SDKs!
Front-end applications are best-suited when you want users to connect their wallets to your web application and interact with your programs using their wallet.
Learn By Example
Check out our live demos to see what you can build on Solana and how to build it.
Create A New Project
The easiest way to get started is by using the CLI.
npx thirdweb create app --solana --ts
You're project is ready to start interacting with your programs, tokens and NFTs on Solana.
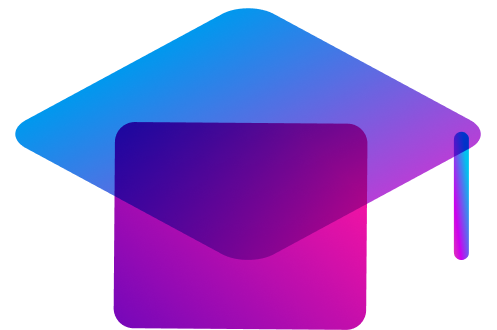
Continue to the Interact with Programs section
Integrate Into Existing Projects
If you have an existing project you want to integrate thirdweb into, install the thirdweb SDKs:
- npm
- Yarn
npm install @thirdweb-dev/sdk @thirdweb-dev/react @metaplex-foundation/js @project-serum/anchor @solana/wallet-adapter-wallets @solana/wallet-adapter-react @solana/wallet-adapter-react-ui
yarn add @thirdweb-dev/sdk @thirdweb-dev/react @metaplex-foundation/js @project-serum/anchor @solana/wallet-adapter-wallets @solana/wallet-adapter-react @solana/wallet-adapter-react-ui
Then wrap your application in the ThirdwebProvider
to get started.
import { ThirdwebProvider } from "@thirdweb-dev/react/solana";
// Change the network to the one you want to use: "mainnet-beta", "testnet", "devnet", "localhost" or your own RPC endpoint
const desiredNetwork = "devnet";
export const MyApp = () => {
return (
<ThirdwebProvider network={desiredNetwork}>
{/* Your App Goes Here */}
</ThirdwebProvider>
);
};
Any page wrapped within the ThirdwebProvider
has access to the features of the React SDK, such as:
useWallet
- Get the current wallet and its stateuseSDK
- Get the current SDK instanceuseProgram
- Get any deployed program instance
This setup is compatible with the Solana Wallet Adapter library. You can use any of the supported wallets.
Existing Wallet Connection Setups
If you already have a connection and wallet setup using Solana's web3js,
you can use the ThirdwebSDKProvider
to access the SDK functions without any of the wallet management functionality.
import { ThirdwebSDKProvider } from "@thirdweb-dev/react/solana";
import { useWallet } from "@solana/wallet-adapter-react";
export const MyApp({ Component, pageProps }) {
const wallet = useWallet();
return (
<ThirdwebSDKProvider network={"devnet"} wallet={wallet}>
{/* Your App Goes Here */}
</ThirdwebSDKProvider>
);
}