Build A Web3 App
Use the CLI to create a web application with the React and JavaScript SDKs pre-configured:
npx thirdweb create app --evm
This will kick off an interactive series of questions to help you get started:
- Give your project a name
- Select
Create React App
as the framework - Select
JavaScript
orTypeScript
as the language
Exploring The Project
The create
command generates a new directory with your project name.
Open this directory in your text editor.
Inside the index.js
file,
you'll find the ThirdwebProvider
wrapping the entire application.
This wrapper allows us to use all of the React SDK's hooks and UI Components throughout the application, as well as configure an activeChain
;
which declares which chain our smart contracts are deployed to.
Since we deployed our smart contract to the Goerli
network, we'll set the activeChain
to "goerli"
:
Interact With Contracts
To connect to your smart contract in the application,
provide your smart contract address (which you can get from the dashboard) to the
useContract
hook like so:
import { useContract } from "@thirdweb-dev/react";
export default function Home() {
const { contract } = useContract("<CONTRACT_ADDRESS>");
// Now you can use the contract in the rest of the component!
}
You can now call any function on your smart contract with useContractRead
and
useContractWrite
hooks.
Each extension you implemented in your smart contract also
unlocks a new functionality for you to use in the SDK.
Let's look at how this works by reading our smart contract's NFTs and then minting new ones.
Reading Data
The NFT Collection contract we built earlier implements the ERC721 and ERC721Supply extensions.
By doing so, we can use the View All
functionality in our application! In the React SDK, that is available
through the useNFTs
hook, which we can use by providing the contract
object to the hook like so:
import { useContract, useNFTs } from "@thirdweb-dev/react";
export default function Home() {
const { contract } = useContract("<CONTRACT_ADDRESS>");
const { data: nfts, isLoading: isReadingNfts } = useNFTs(contract);
}
Displaying Data
Use the NFT Media Renderer component to
render each of the NFTs loaded from the useNFTs
hook, making use of the loading state in a conditional statement:
import { useContract, useNFTs, ThirdwebNftMedia } from "@thirdweb-dev/react";
export default function Home() {
const { contract } = useContract("<CONTRACT_ADDRESS>");
const { data: nfts, isLoading: isReadingNfts } = useNFTs(contract);
return (
<>
{isReadingNfts ? (
<p>Loading...</p>
) : (
<div>
{nfts?.map((nft) => (
<ThirdwebNftMedia metadata={nft.metadata} key={nft.metadata.id} />
))}
</div>
)}
</>
);
}
Writing Transactions
Since our smart contract implements the ERC721Mintable
extension,
the Mint feature is available to us in the SDK.
Use the Web3Button
component to perform a mint
transaction; which ensures the user
has their wallet connected and is on the right network before calling the function:
// Place this import at the top of the file
import { Web3Button } from "@thirdweb-dev/react";
// ...
// Place this beneath your existing NFT display logic:
<Web3Button
contractAddress={"<CONTRACT_ADDRESS>"}
action={(contract) =>
contract.erc721.mint({
name: "Hello world!",
// Place any URL or file here!
image: "<your-image-url-here>",
})
}
>
Mint NFT
</Web3Button>;
Deploy Your App
To host your application on IPFS and share it with the world, run the following command:
yarn deploy
That's it! 🥳 This command uses Storage to:
- Create a production build of your application
- Upload the build to IPFS
- Generate a URL where your app is permanently hosted.
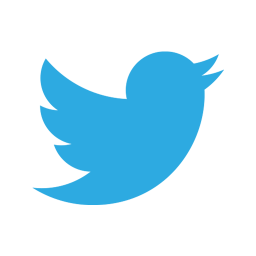
Share your app with us on Twitter!
What's Next?
Congratulations on making it this far! 🎉
Now you've learned the basics, take your skills to the next level by building on top of our templates.