Deploy A Smart Contract
You may want to build a smart contract from the ground up, or deploy a prebuilt solution that meets your needs; we provide solutions for creating and deploying any smart contract.
Begin Your Journey
Select which card best describes your contract needs below.
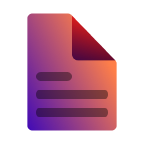
Explore
Secure, gas-optimized, and audited contracts that are ready to be deployed with one-click.
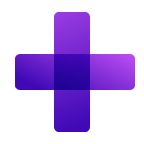
Build your own
Create custom contracts that are specific to your use case using ContractKit and Solidity.
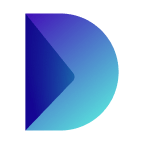
Deploy from source
Already have a contract ready to deploy? Learn how to use our interactive CLI to ship it.
Creating A Project
We can use the CLI to create a new project with a smart contract inside, and ContractKit installed for us.
npx thirdweb create contract
This will kick off an interactive series of questions to help you get started:
- Give your project a name
- Select
Hardhat
as the framework - Select
ERC721
as the base contract - Select
None
for optional extensions
Exploring The Project
The create
command generates a new directory with your project name.
Open this directory in your text editor.
Inside the contracts
folder, you'll find a Contract.sol
file; this is our smart contract written in Solidity!
If we take a look at the code, you can see that our contract is inheriting the functionality of ERC721Base, by:
- Importing the contract
- Inheriting the contract; by declaring that our contract
is ERC721Base
- Implementing any required methods such as the
constructor
.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@thirdweb-dev/contracts/base/ERC721Base.sol";
contract Contract is ERC721Base {
constructor(
string memory _name,
string memory _symbol,
address _royaltyRecipient,
uint128 _royaltyBps
) ERC721Base(_name, _symbol, _royaltyRecipient, _royaltyBps) {}
}
This inheritance pattern lets us use functionality from other contracts inside of ours, modify it, and add custom logic.
For example, our contract currently implements all of the logic inside the ERC721Base.sol contract; which implements the ERC721A standard with several useful extensions.
Adding Extensions
Extensions are a great way to add individual pieces of functionality to your contract, such as Permissions.
You can follow the same pattern as we did with the base contract to add an extension:
Import
import "@thirdweb-dev/contracts/extension/PermissionsEnumerable.sol";
Inherit
contract Contract is ERC721Base, PermissionsEnumerable {
// ...
}
Implement
contract Contract is ERC721Base, PermissionsEnumerable {
constructor(
string memory _name,
string memory _symbol,
address _royaltyRecipient,
uint128 _royaltyBps
) ERC721Base(_name, _symbol, _royaltyRecipient, _royaltyBps) {
// Give the contract deployer the "admin" role when the contract is deployed.
_setupRole(DEFAULT_ADMIN_ROLE, msg.sender);
}
// Example: Only allow the "admin" role to call this function.
function helloWorld() external onlyRole(DEFAULT_ADMIN_ROLE) {
// ...
}
}
That's it! 🥳 You just made an ERC721A NFT collection smart contract with permission controls!
Deploying
Let's deploy our contract to the blockchain, by running the following command:
yarn deploy
That's it! 🥳 This command does the following:
- Compiles your contract
- Uploads your contract source code (ABI) to IPFS
- Opens the dashboard for you to select one of our supported networks to deploy to
First, we need to enter the values for our contract's constructor:
_name
: The name of our contract_symbol
: The symbol or "ticker" given to our contracts tokens_royaltyRecipient
: The wallet address that will receive the royalties from secondary sales_royaltyBps
: The basis points (bps) that will be given to the royalty recipient for each secondary sale, e.g. 500 = 5%
Finally, select the network you want to deploy to (we recommend the Goerli test network), and click "Deploy Now".
Once your contract is deployed, you'll be redirected to your contract's dashboard:
Explore Contracts
From the explore page, you can choose from a curated collection of gas-optimized, audited, and battle-tested smart contracts built by trusted open-source protocols and the thirdweb team; ready to deploy in one click.
For example, let's select the NFT Collection smart contract built by the thirdweb team:
From this page, we can discover the contract's features, view its source code, see which extensions it implements, and deploy it to the blockchain of our choice.
Click the "Deploy Now" button to enter the deployment flow, and enter the metadata of your smart contract; such as its name, symbol, image and description:
Finally, select the network you want to deploy your smart contract to and click "Deploy Now":
Once your contract is deployed, you'll be redirected to your contract's dashboard:
Deploy Your Smart Contract
Use the CLI to deploy your smart contract to the blockchain.
From the same directory as your .sol
smart contract file, run the following command:
npx thirdweb deploy
That's it! 🥳 This command does the following:
- Compiles your contract
- Uploads your contract source code (ABI) to IPFS
- Opens the dashboard for you to select one of our supported networks to deploy to
Open the generated URL in your browser, and populate the fields of
your smart contract's constructor
.
Finally, select the network you want to deploy to (we recommend the Goerli test network), and click "Deploy Now".
Once your contract is deployed, you'll be redirected to your contract's dashboard: